Project: Weaver
Weaver is a desktop address book application meant for NUS students. It helps to manage and store information about your friends and family. In particular Weaver helps students maintain their day to day school timetable and schedule their daily reminders so that they can keep up with the fast paced university life. It also includes social media integration and other features that is in sync with students of NUS.
The user interacts with it using a Command Line Interface, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC. Some of the tools used for this project are Github, Intellij, SceneBuilder and JAVAFX.
Code contributed: [Functional code] [Test code]
FaceBook Integration since v1.3
This feature helps you view the profile page of a person in your contact list. This way we can know more about your friends
and colleagues.
Below are listed some conditions for the command.
-
The index of the person must be valid, that is it must be a positive integer and within bounds.
-
The person must have the username he/she uses on Facebook.
-
You will have to log in to your own account before you view the profile page of the person.
Command Format - Facebook [INDEX]
e.g. - facebook 1
Result - The facebook homepgae shows up on the browser panel.
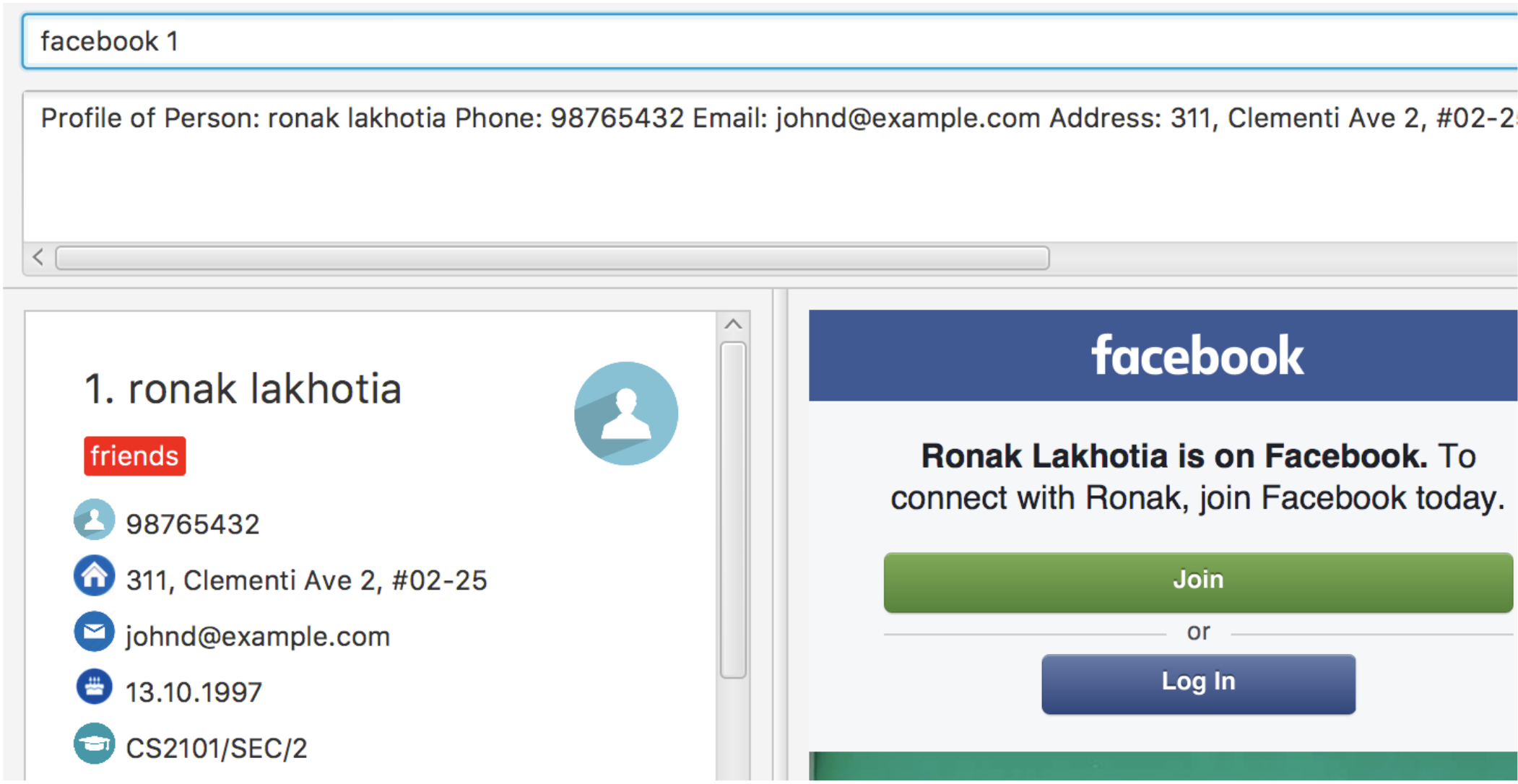
-
If the person does not have a facebook account or the username is not entered in Weaver, you will be notified.
e.g. - facebook 2
Result - You will see a prompt telling you that the person has no username.

End of Extract
Justification
-
This implementation is very useful for users as they can connect with their friends and family through Weaver, thereby saving them the trouble of opening a browser on their desktop. It also promotes a healthy social media life.
-
The command format is easy to follow. Opening the facebook page on the browser panel is more convenient than searching for the person manually on the default browser.
FaceBook Integration
-
The facebook command helps the user to view the profile page of a person on the contact list.
-
If the user has no username the facebook page will not be loaded in the browser panel and an exception will be thrown.
-
The command takes in one argument which is the index of the person.
Working flow
-
The
FaceBookCommand Parser
class parses the argument and throws an exception if the index entered is invalid. -
A new event
handleFaceBookEvent
is then raised. -
The Brower Panel class then loads the page with the url of the profile page to be viewed on Facebook.
Below are few code snippets to guide you through the working of the feature.
public void loadPersonFaceBookPage(ReadOnlyPerson person, String username) {
String url = FACEBOOK_PROFILE_PAGE + username; loadPage(url); } ---
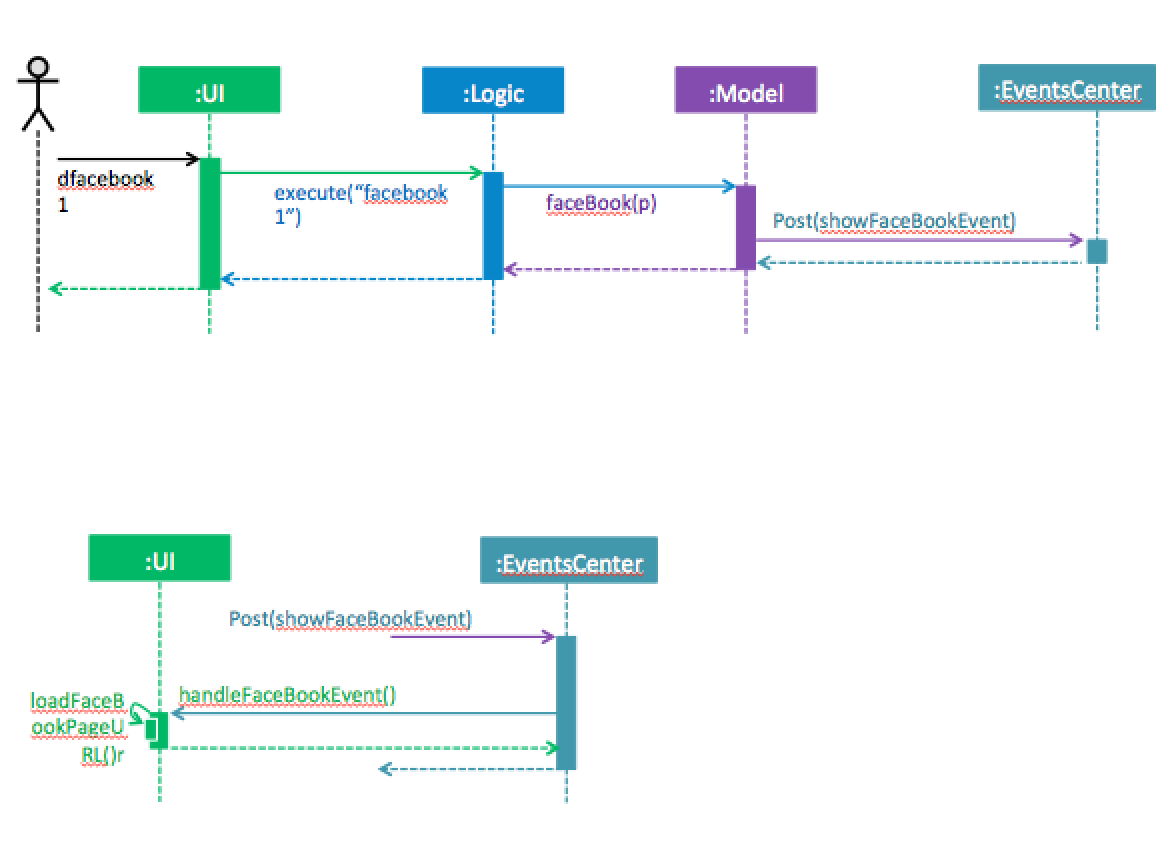
Figure 3.1
-
Refer to figure 3.1 above which shows the sequence diagram of the Facebook command.
It clearly demonstrates how the command interacts with the Logic, Model and UI components.
It also shows how the event is raised and hence the page is loaded in the browser panel.
Reasons for this kind of implementation
-
This helps the user to take a quick glance of the the profile image of the contacts on his/her list.
-
It is better than opening a brower on the desktop.
-
It does not require the user to search for the person on the facebook homepage which can be a tedious task.
Design Considerations
Aspect: How should the user view the profile page.
Alternative1 : View the profile page on a web browser
Pros: Helps user exercise other functionalities like using messenger.
Cons: Does not make use of Weaver in any useful way.
Alternative2: View the profile page on the browser panel.
Pros: Makes it quicker and adds a function to the personalised Weaver.
Cons: Limits the functionalities that can be used on facebook.
End of Extract
Add Photo to contacts : photo
Since v1.2
-
Adds a Display picture to the contact.The image file must be present in your PC.
-
Each person in your contact list can have atmost one display picture.
-
You can change the display picture of a person by specifying the filepath of another image.
-
The person must have a display picture for the delete operation to work else Weaver will notify you that the delete operation is not valid.
-
Once the Image is set and the file is then removed from the specified directory, Weaver will no longer display the picture.
-
The command also follows the undo/redo mechanism.
e.g. Photo 1 /Users/ronaklakhotia/Desktop/Ronak.jpeg
adds the image Ronak.jpg
to the contact with
index 1 in the address book.
-
Result - Picture is added to the person at index 1.
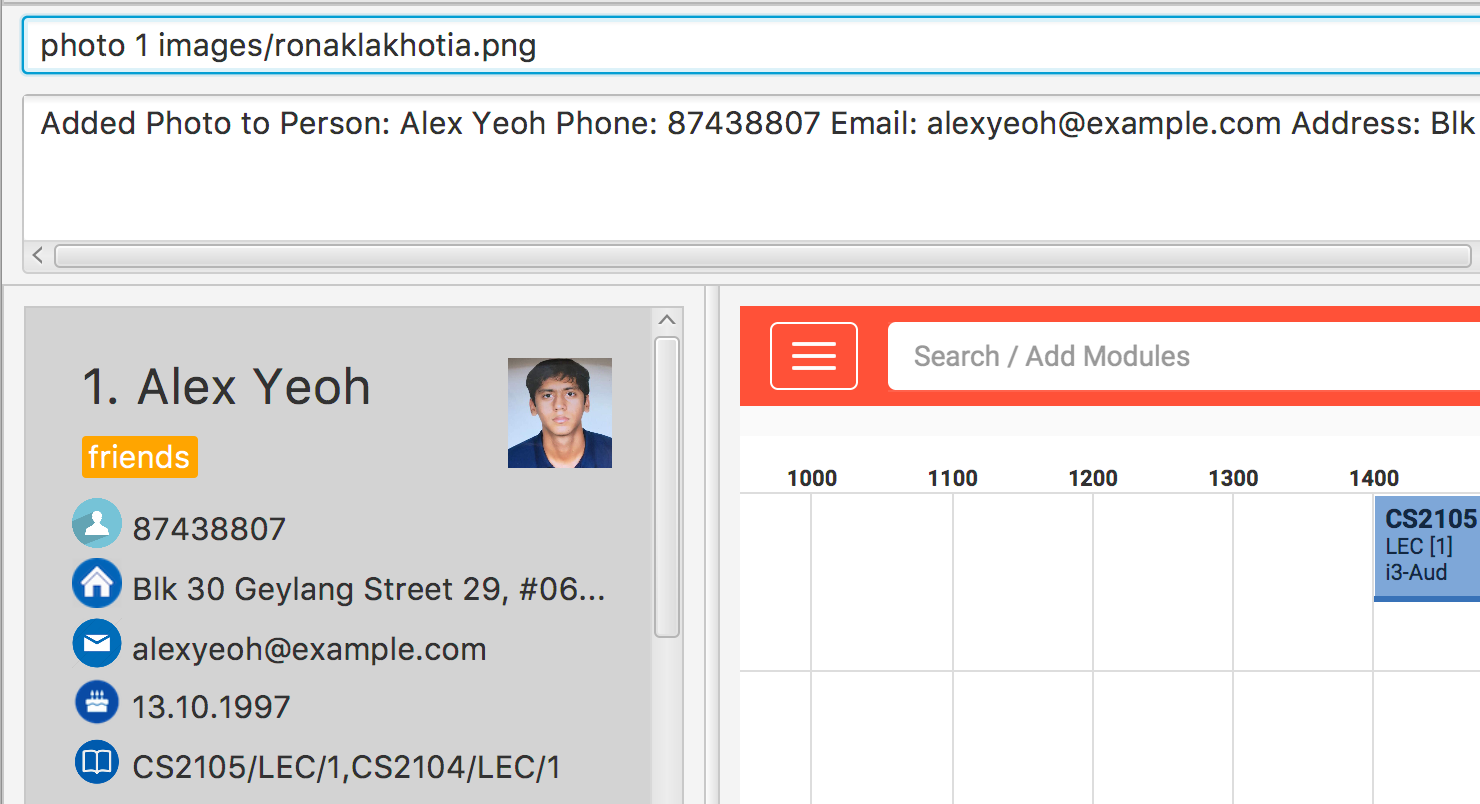
e.g. Photo 1 delete
.
-
Result - Picture is deleted from the person at index at 1.

Different Scenarios :
End of Extract
Justification
-
Allows user to choose any image in their local device and use Command Line Interface to specify the path of the image.
-
This way the user can display the pictures on their contact lists. Users can also identify people more easily if two or+ more people have the same name. It also adds a visual appeal to the Application.
Adding a photo to a contact in your personalised Weaver.
-
The add Photo command adds a display picture to a particular contact.
-
The command takes in two parameters, TargetIndex that is the index of the person to add a photo and the image filepath.
-
The command can also be used to delete an existing picture of a person.If the person has no display picture and the user attempts to delete a picture, an exception will be thrown.
-
The photo Command is inherited from the
UndoableCommand
class. The command supports the Undo/Redo mechanism in Weaver. -
The photo command is done with the help of ImageView property in JAVAFX, hence it is an attempt to enhance the UI.
PRE-REQUISITE
There is a pre-requisite for the command to work. The image that is going to be added to the contact must already be
present on the user’s local PC. If it is not so, Weaver is going to throw an exception and prompt the user to add a valid File path.
Working Flow
-
The
PhotoCommandParser
Class parses the arguments entered in the photo command.The class then throws an Exception with the appropriate message depending on whether the File name is present in the path specified by the user or if the number of arguments entered is not equal to two. -
The
PhotoCommand
class takes care of Invalid conditions like incorrect index. -
It then creates a new instance of the
FileImage
class.FileImage
is an attribute that every person must have. A newPerson
is created with the updatedFileImage
and the existing model is updated.
Sequence Diagram
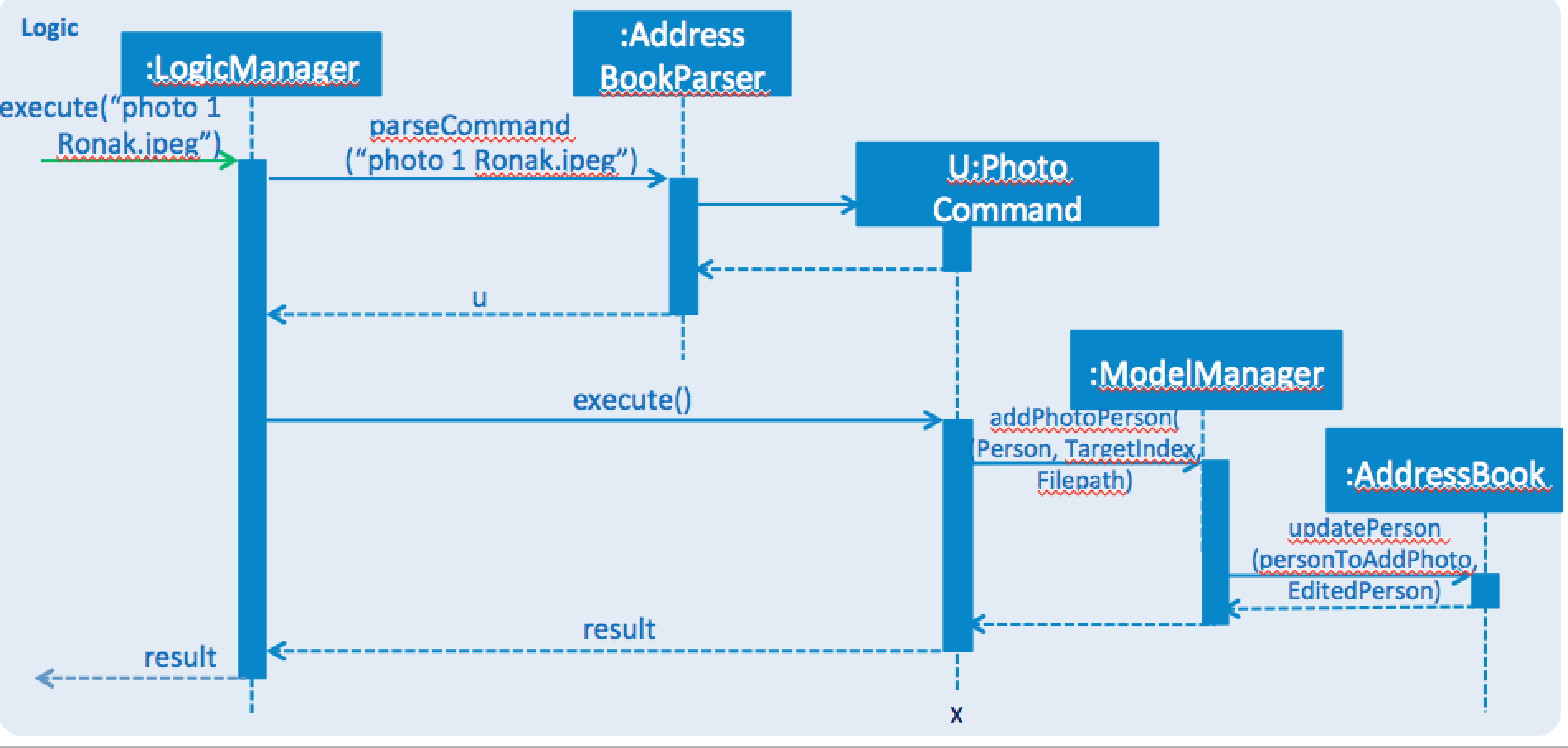
Figure 3.2
The Sequence diagram (Figure 3.2) above shows the working flow of the Photo command feature.
-
The
ModelManager
class implements theaddPhotoToPerson
method that adds a valid file image as an attribute to the person.
person.imageProperty().setValue( new FileImage(FilePath)); updateFilteredPersonList(PREDICATE_SHOW_ALL_PERSONS); indicateAddressBookChanged();
-
The filePath entered must be a valid one, else Weaver will throw an exception.
if (FileExists) { try { Index index = ParserUtil.parseIndex(keywords[0]); return new PhotoCommand(index, (keywords[1])); } catch (IllegalValueException ive) { throw new ParseException( String.format(MESSAGE_INVALID_COMMAND_FORMAT, PhotoCommand.MESSAGE_USAGE)); } }
-
Once the Image is set and the file is then removed from the specified directory, Weaver will no longer display the picture.
Refer to Figure 3.3 below for the Inheritance diagram of the command.
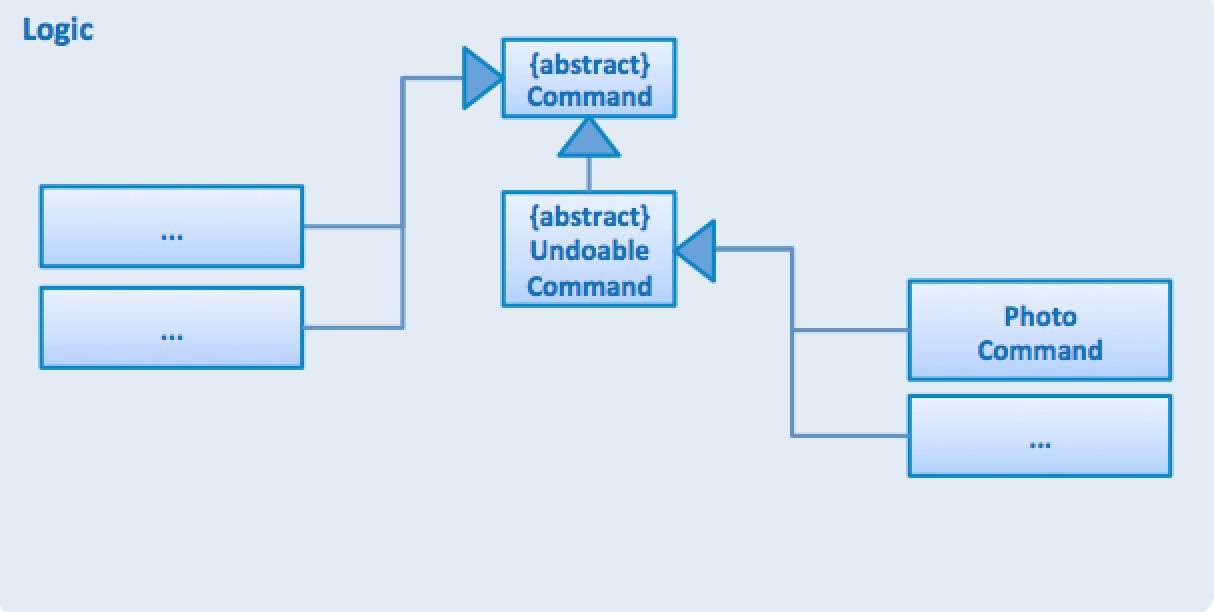
Figure 3.3
The binder for attaching the image to the Person Card each time, is implemented in the following way.
Image display = new Image(file.toURI().toString()); image.setImage(display);

Figure 3.4 Wireframe
The wireframe for binding the image is shown above in Figure 3.4.
Reasons for this type of implementation
-
The feature can be implemented using CommandLine by just stating name of the file.
-
The index can be used easily used to reference the person.
-
The user can access the image from any directory in the PC.
Design considerations
Aspect: How should the user choose the Image.
Alternative1: (current choice): Enter the File path present on
the desktop.
Pros: Makes use of Commad Line Interface.
Cons: User has to make sure the file exists on his/her Desktop.
Alternative2: Make use of FileChooser that prompts user to choose file from
any directory.
Pros: User can choose any image from any location.
Cons: Makes use of a dialog box instead of Command Line.
Aspect: How should the image be stored.
Alternative1: Image is stored in the images
directory of the project by invoking ImageStorage
class.
Pros: Each image is assigned a unique hashcode.
Cons: The working flow is not easy for incomimg developers to follow.
Alternative2: Image should be stored in the directory whose path is satisfied.
Pros: Easy for new developers to comprehend.
Cons: If the image is removed from the directory, Weaver will have no access to the image.
End of Extract
Sending Emails to your contacts : email
since v1.5
Weaver now allows you to send emails to all your contacts with a common tag!
If you want to send an important mail to people who are tagged as temmates, you are just a command away!.
This command will open the default browser in your desktop and direct you to your gmail account with the
send to recipients
and Subject line filled in.
Format: email t/[TAG] s/[SUBJECT]
.
You must have a Gmail Account to send mails. |
-
You cannot include more than one tag. Also you must specify 1 tag
-
The tag specified must be attached to atleast one person in Weaver.
-
You must specify a valid subject line for the mail.
e.g. email t/friends s/birthday party
Result
Email is sent!

e.g. email t/friends t/colleagues s/subject
Result
Multiple tags cannot be entered

End of Extract
Justification
-
Gmail is the most widely used Email service platform and everyone around has a gmail account.
-
Multiple tags are not allowed because the email that a user may want to send to his/her friends will not be the same he would want to someone to whom he owes money.
Email Feature
-
Weaver includes the
email
command which helps users contact persons on their contact list using the default browser in their desktop. -
This feature will work for users with a Gmail Account.
The recipients are those persons with a common tag. |
-
The command takes in two parameters, the tag name and the subject line that the user wants in the email body.
Refer to the sequence diagram 4.1 below to see how the command parses the arguements and completes execution.

Figure 4.1
The command will throw an exception in the following cases :
-
The number of tags entered is not equal to 1.
-
The tag name does not match any of the tags in Weaver.
-
The subject line is not valid.
-
Below is the high level sequence diagram of the command.

The following code snippet shows the Gmail Browser URL used and how the desktop browser is opened.
appendEmailAddress = appendEmailAddress.substring(0, appendEmailAddress.length() - 1);
String Gmail_Url = "https://mail.google.com/mail/?view=cm&fs=1&to=" + appendEmailAddress + "&su=" + subject;
if(Desktop.isDesktopSupported()) { Desktop.getDesktop().browse(new URI(Gmail_Url)); }
Reasons for this kind of implementation
-
Gmail is the most widely used web browser and most people are likely to have a Gmail account. Hence Gmail was the most appropriate email service.
-
Opening the Gmail compose page on the browser is more convenient than working on the browser panel of Weaver since the browser panel restricts some functionalities.
Design Considerations
Aspect: How should the user send the mail.
Alternative1 : (currently used) Open the default web browser
Pros: Makes sending emails a hassle free job and this also makes use of Weaver CLI in a good way.
Cons: Depends on the type of browser and its compatibility
Alternative2: Send the email using browser panel
Pros: Makes it quicker and adds a function to the personalised Weaver.
Cons: Limits the functionalities that can be used on Gmail since the browser panel is not updated.
End of Extract
Reminders Panel since v1.4
Have you ever felt the need to be constantly reminded of your daily assignments. Fret not! Weaver helps you keep up to date with your daily school assignments. Just add your reminders using a single command and weaver will display your remindersdepicting the priority level of that assignment and the due date.That way, you can keep scrolling through your reminders panel when you open the application and be aware of the upcoming tasks.
There are three basic commands.
-
Add a reminder to your list.
Command Format -Reminder g/DETAILS p/PRIORITY OF TASK d/DUEDATE
e.g. `Reminder g/CS2103T Assignment p/High d/12.11.2017 -
Result
The reminder is added to your reminders panel.

-
Remove a reminder from your list.
Command Format -Remove [INDEX OF REMINDER]
e.g.Remove 1
-
Result
The reminder is removed from your reminders panel.

-
Change an existing reminder.
Command Format -Change [INDEX] field to be changed
e.g.change 1 d/22.12.2017
The above command will change the due date of the reminder to the new date. -
Result
The reminder duedate is changed .

End of Extract
Justification
-
A reminders panel is very important in a students day to day life as it helps them keep up with the daily tasks and assignments.
-
The implementation is easy and the UI is clean without any attempt to overcomplicate it.
Adding a reminders list to Weaver.
-
The reminders panel displays the list of reminders that the user has entered.
-
A reminder class has three main attributes, Details of reminder, due date and the priority level of the reminder.
-
Reminder objects are kept in the UniqueReminderList which ensures there are no duplicate reminders.
-
The Model manager carries out the operations related to reminders.
-
Exceptions are thrown if invalid commands entered.
-
There are three main commands associated with this feature.
-
Adding a reminder to the list.
e.g. -reminder g/CS2103T Assignmet p/High d/12.11.2017
. -
Removing a reminder.
e.g. -remove 1
. -
Changing a reminder.
e.g. -change 1 d/12.12.2017
.
-
-
The commands to add and remove reminders will inherit from Undoable Command.
The diagram (Figure 5.1) below gives the high level architecture diagram of the implementation.

-
The reminders panel is implemented in a similar manner like the person list panel.
-
Data is written/stored in the same addressbook.xml file.

(Figure 5.2)
Figure 5.2 above shows the Logic Component Diagram of the Reminders Panel implementation.
Note how the LogicManager class implements the interface Logic and is associated with the undo redo stack. |
Reasons for this kind of implementation
-
The implementation is easy to follow and comprehend.
-
Storing in the same file makes the organization better.
Design Considerations
Aspect: How to store each reminder
Alternative1 : (currently used) Reminders stored in the addressbook.xml file
Pros: Implementation easy to follow for future developers.
Cons: Can violate higher levels of software engineering principles.
Alternative2: Store reminder objects separately in a different xml file
Pros: More in sync with SE principles.
Cons: Harder to implement and follow.
End of Extract
Enhancements Proposed: v2.0 Iteration
Listed below are some of the enhancements I intend to do in Weaver by the v2.0 iteration.
Favourites list Feature:
This feature will display all persons in Weaver that are frequently accessed based on the commands.
Say for instance, a person’s location is searched a few times and that person is also looked up on facebook,
it will increase the popularity of the person and he/she will show up on the top of the list.
External Behavior:
The method that can be used for this implementaion is to keep another attribute, called popularity for each person.
This attribute is incremented each time a person is accessed. Depending on the value of this attribute the list is to be sorted.
Justification
-
Contacts who are frequently looked up in Weaver are going to be close relatives and friends of the user. Hence It is justified to separate contacts based on popularity.
-
The method of implementation would be to raise an event each time a person is accessed. This will in turn also raise an event to indicate that the addressbook has changed.
Implementation
Refer to figure 5.3 below that gives a brief outline of how I plan to implement the popularity list.

Figure 5.3
Other Significant Contributions Made in Weaver and this Module in general.
Code contributions besides features stated above.
-
Implemented Case-insensitive find command. This is in harmony with Software Engineering principles as the end product is easier to use for users. So a command like
fINd
will be executed nevertheless. -
Added an important attribute, Date Of Birth to each person. (Pull requests #17).
-
Implemented a Search Command. The search command makes the find command more powerful as it searches for a person based on his/her name and Date of Birth. It is useful when there are more than one perosn with the same name and the user wants to look only for a particular person (Pull requests #116).
-
Wrote additional tests to increase coverage (Pull requests #8) .
-
Implementing the Reminders panel was very useful as it gave me a good understanding of how the various components of the codebase interact with each other.
-
Wrote extensive use cases and documentation in the form of user guide and developer guide.
Active Participation in Forum.
-
I have participated actively in forum discussions and have helped my peers. Below are two such instances.
1) I helped a colleague of mine resolve a issue with code collation. I was credited by the person who
asked the question. (Here) is the link to the issue.
2) I also engaged in a healthy discussion with my peers about Single Responsibility Principle and helped the person
by giving me insight. (Here) is the link to the issue.
Technical Leadership
-
I have demonstrated good coding and technical skills by helping one of my peers find an important bug and also offered my code for reuse.
1) I helped my friends resolve a bug that seemed difficult to get around with. It took a long time to come up with a solution and I was credited
for the issue being resolved. (Here) is the link to the issue.
2) I also offered my code for reuse. The feature I proposed was reminders panel implementation.
(Here) is the link to the issue.
3) I helped other teams find bugs in their product during the practical exam simulation we had in Week 11.
(Bug) is the link to the issue.
4) In the team project, I took a leader’s role and created issues, therby assigning deadlines.
5) Also set up important tools for the project like Travis and Coveralls.
PROJECT: NUSEvents
-
I was actively involved in a side project of my own over the recent summer break.
ABOUT THE PROJECT
Students coming into university want to experience an enriching and diverse campus life. One of the most integral parts of a campus life is the host of events taking place around the campus. We want to bring forth an application by means of which members of the NUS family will be able to view the various events going around the campus on a day to day basis. Now there will be no need to worry about searching websites, scrolling through emails and pages on Facebook to know what events are being organized by various faculties and clubs. This will be convenient for all students and members of the various faculties as it will enable them to indicate their interest in the events that they want to attend.
-
The project was about developing an Android Application that will help students keep track of the various events going around campus.
-
The project was done with another friend of mine and we were careful in following the Software Engineering norms. (Here) is a link to the project repository.